Hero Components
A collection of beautiful hero sections with various styles and features.
Hero Section Variants
Our starter includes two pre-built hero section layouts that you can use in your project:
- Minimal Layout (
layoutVariant: 'default'
) - A clean, focused design with essential elements - Featured Layout (
layoutVariant: 'featured'
) - Enhanced layout with prominent visuals and content
Before you start, make sure you have a Hero.astro
file inside your
sections folder. If you donβt have it, create one and copy the code. If you already have it, you can just overwrite the content with
the code.
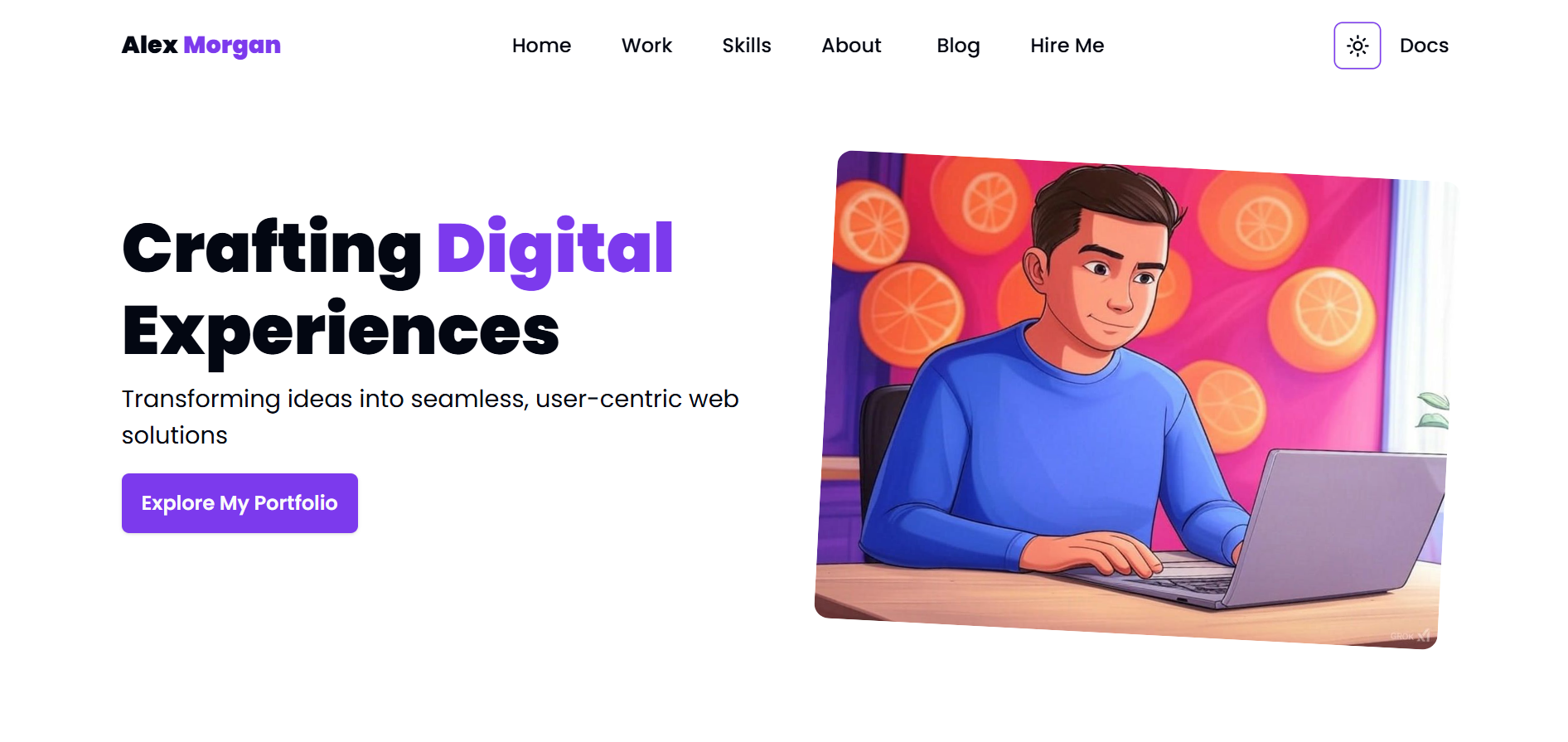
---import { Button } from '@/components/ui/button';import { getHeroByVariant } from '@/lib/helper';import { getHeroContent } from '@/lib/queries';import type { HeroButtonProps } from '@/lib/types';import { checkImageExists } from '@/lib/helper';import { Image } from '@unpic/astro';
const hero = await getHeroContent();
// Get specific hero variantconst defaultHero = getHeroByVariant(hero, 'default');
// Add fallback in case the variant doesn't existif (!defaultHero) { throw new Error('Default hero variant not found in Sanity content');}
// Clean up the strings and split the title into wordsconst cleanText = (text: string) => text .replace(/[^\x20-\x7E]/g, ' ') .replace(/\s+/g, ' ') .trim();const title = cleanText(defaultHero.title || '');const accentWord = cleanText(defaultHero.titleAccent || '');
// Split title into words and map them with accent stylingconst words = title.split(' ');---
<section id="home" class="pt-10 md:pt-0"> <div class="relative z-40 text-foreground"> <div class="container flex h-screen flex-col items-start justify-center md:px-0" > <div class="mx-auto max-w-[1200px] md:grid md:grid-cols-2 md:items-center md:gap-16" > <div class="mb-8 mt-[7rem] md:my-0"> <h1 class="mb-2 text-500 font-900 capitalize leading-[1.2] md:text-600 lg:text-750" > { words.map((word, index) => word.toLowerCase() === accentWord.toLowerCase() ? ( <span class="text-primary">{word}</span> ) : ( <span> {word} {index < words.length - 1 ? ' ' : ''} </span> ) ) } </h1> <p class="mb-4 md:text-200"> {defaultHero.subtitle} </p> { defaultHero.button.map((item: HeroButtonProps) => ( <a href={item.url}> <Button className="py-6 font-600 capitalize"> {' '} {item.title}{' '} </Button> </a> )) } </div>
<div class="w-full duration-300 ease-in md:mt-20 md:rotate-3 lg:hover:rotate-0" > { defaultHero.imageType === 'upload' && checkImageExists(defaultHero.heroImage?.asset.url) ? ( <Image class="w-full rounded-xl object-cover" src={defaultHero.heroImage?.asset.url as string} alt={defaultHero.heroImage?.alt} background="blurhash" /> ) : defaultHero.imageType === 'url' && checkImageExists(defaultHero.heroUrl) ? ( <img class="w-full rounded-xl object-cover" src={defaultHero.heroUrl} alt={defaultHero.heroImage?.alt} /> ) : null } </div> </div> </div> </div></section>
import { useEffect, useState } from "react";import type { HeaderData } from "@/lib/types";import Nav from "@/components/Nav";import { ModeToggle } from "@/components/ModeToggle";import { AlignLeft, X } from "lucide-react";
interface HeaderProps { header: HeaderData[]; isBlogDetailsPage?: boolean;}
const Header = ({ header, isBlogDetailsPage }: HeaderProps) => { const [isClicked, setIsClicked] = useState(false); const [scrolling, setScrolling] = useState(false);
const toggleNavClick = () => { setIsClicked(!isClicked); };
// Change header background color on scroll useEffect(() => { const handleScroll = () => { const scroll = window.scrollY;
if (scroll > 100) { setScrolling(true); } else { setScrolling(false); } };
window.addEventListener("scroll", handleScroll);
// Cleanup the event listener on component unmount return () => { window.removeEventListener("scroll", handleScroll); }; }, []);
// Clean up the strings and split the title into words const cleanText = (text: string) => text .replace(/[^\x20-\x7E]/g, " ") .replace(/\s+/g, " ") .trim(); const title = cleanText(header[0].logo.text || ""); const accentWord = cleanText(header[0].logo.textAccent || "");
// Split title into words and map them with accent styling const words = title.split(" ");
const linkColorClass = isBlogDetailsPage && !scrolling ? "text-white" : scrolling ? "text-foreground" : "text-foreground dark:text-white";
return ( <header className={`${scrolling ? "bg-background shadow-xl duration-500 animate-in" : "duration-500 animate-out"} fixed top-0 z-[500] w-full text-foreground`} > <div className="container flex items-center justify-between py-4 md:px-0"> {/* Logo */} <div className="z-10 text-200 font-900"> {header[0].logo.logoType === "text" ? ( <a className={linkColorClass} href="/"> {words.map((word, index) => ( <div key={index} className="inline-block"> {word.toLowerCase() === accentWord.toLowerCase() ? ( <span className="mx-1 text-primary">{word}</span> ) : ( <> {word} {index < words.length - 1 && " "} </> )} </div> ))} </a> ) : ( <a href="/"> <img src={header[0].logo.image?.asset.url} alt="Logo" /> </a> )} </div>
{/* Navbar */} <Nav scrolling={scrolling} navigation={header[0].navigation} isClicked={isClicked} toggleNavClick={toggleNavClick} isBlogDetailsPage={isBlogDetailsPage} />
<div className="z-10 flex items-center gap-4"> <ModeToggle />
{/* Menu buttons */} <div className={`${linkColorClass} ml-4 inline-block md:hidden`} onClick={toggleNavClick} > {isClicked ? ( <button className="text-300"> <X name="Close Menu" className="translate-y-1" cursor="pointer" size={28} /> </button> ) : ( <button className="text-300"> <AlignLeft className="translate-y-1" name="Open Menu" cursor="pointer" size={28} /> </button> )} </div> </div> </div> </header> );};
export default Header;
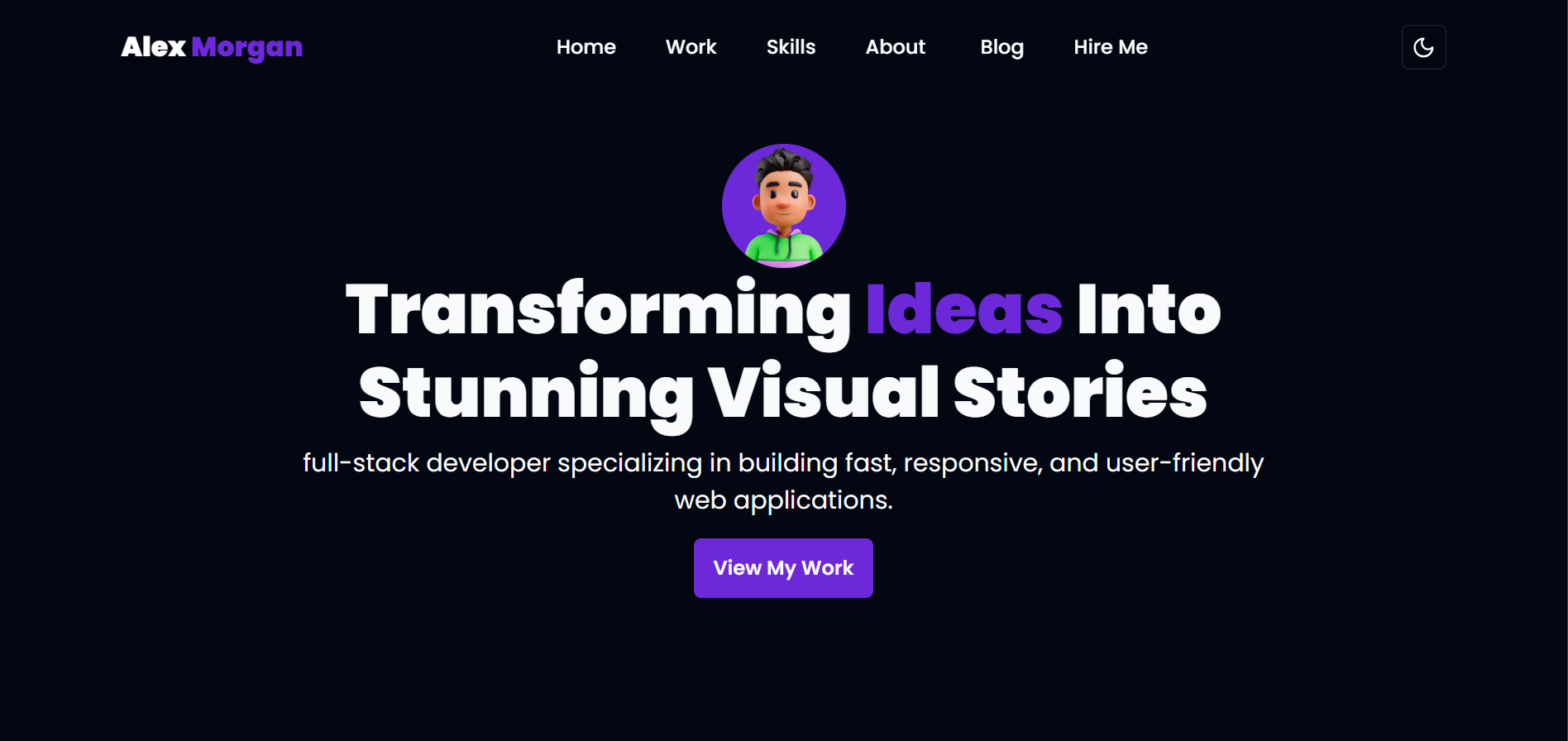
---import { Button } from '@/components/ui/button';import { getHeroByVariant } from '@/lib/helper';import { getHeroContent } from '@/lib/queries';import type { HeroButtonProps } from '@/lib/types';import { Image } from '@unpic/astro';
const hero = await getHeroContent();
// Get specific hero variantconst featuredHero = getHeroByVariant(hero, 'featured');
// Add fallback in case the variant doesn't existif (!featuredHero) { throw new Error('Creative hero variant not found in Sanity content');}
// Function to check if an image URL existsconst checkImageExists = (url: string | undefined): boolean => { return !!url;};
// Clean up the strings and split the title into wordsconst cleanText = (text: string) => text .replace(/[^\x20-\x7E]/g, ' ') .replace(/\s+/g, ' ') .trim();const title = cleanText(featuredHero.title || '');const accentWord = cleanText(featuredHero.titleAccent || '');
// Split title into words and map them with accent stylingconst words = title.split(' ');---
<section id="home" class="pt-6"> <div class="relative z-40"> <div class="container flex h-screen items-center justify-center text-center" > <div class="z-40 max-w-[800px]"> <div class="mb-2"> { featuredHero.imageType === 'upload' && checkImageExists(featuredHero.heroImage?.asset.url) ? ( <Image class="mx-auto size-[100px] rounded-full bg-primary object-cover" src={featuredHero.heroImage?.asset.url as string} alt={featuredHero.heroImage?.alt || ''} width={100} height={100} background="bg-primary" /> ) : featuredHero.imageType === 'url' && checkImageExists(featuredHero.heroUrl) ? ( <img class="mx-auto size-[100px] rounded-full bg-primary object-cover" src={featuredHero.heroUrl} alt={featuredHero.heroImage?.alt || 'Hero Image'} /> ) : null } </div> <h1 class="mb-2 text-500 font-900 capitalize leading-[1.2] md:text-700 lg:text-750" > { words.map((word, index) => word.toLowerCase() === accentWord.toLowerCase() ? ( <span class="text-primary">{word}</span> ) : ( <span> {word} {index < words.length - 1 ? ' ' : ''} </span> ) ) } </h1> <p class="mb-4 md:text-300"> {featuredHero.subtitle} </p> { featuredHero.button.map((item: HeroButtonProps) => ( <a href={item.url}> <Button className="py-6 font-600 capitalize"> {' '} {item.title}{' '} </Button> </a> )) } </div> </div> </div></section>
How to Use
To implement these hero components:
- Copy the code from your desired hero variation
- Paste the code inside
Hero.astro
file inside thesrc/sections
folder - Customize the content as needed through your Sanity Studio
The layout variants are designed to be flexible and work across different types of content while maintaining consistency in your design system.