Project Components
A collection of beautiful work sections with various styles and features.
Project Section Variants
Our starter includes multiple work section layouts, allowing you to switch between different styles.
Before you start, make sure you have a Project.astro
file inside your
sections folder. If you donβt have it, create one and copy the code. If you already have it, you can just overwrite the content with
the code.
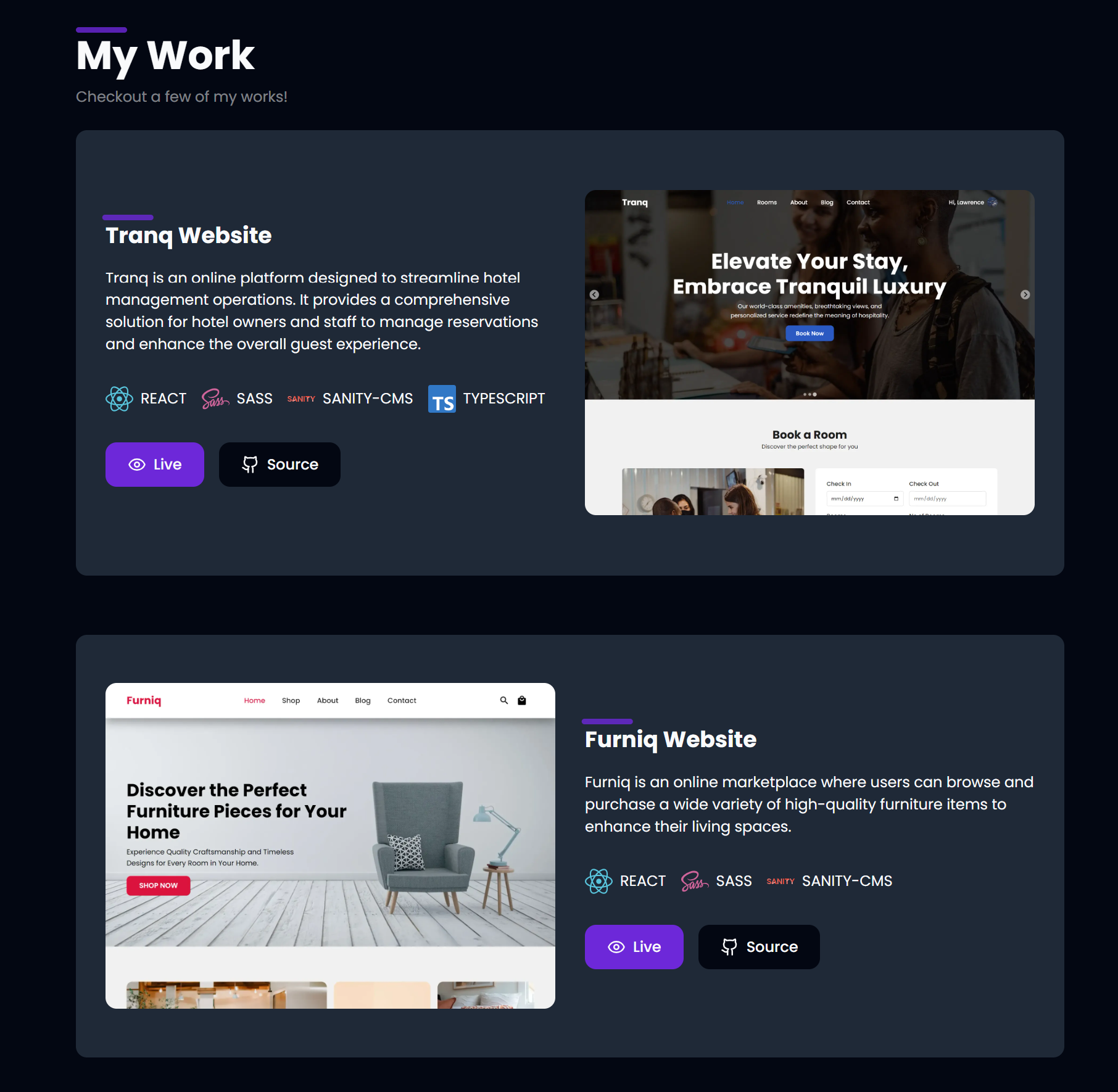
---import { getProjectContent } from '@/lib/queries';import { Button } from '@/components/ui/button';import { Eye, Github } from 'lucide-react';import { checkImageExists, getWorkByVariant } from '@/lib/helper';import Title from '@/components/Title';import { Image } from '@unpic/astro';
const projectsSection = await getProjectContent();
// Get specific hero variantconst defaultProject = getWorkByVariant(projectsSection, 'default');
if (!defaultProject) { throw new Error('Default hero variant not found in Sanity content');}---
<section id={defaultProject.id} class="pt-32 md:pt-[7.5rem]"> <div class="container md:px-0"> <Title title={defaultProject.sectionTitle} subtitle={defaultProject.sectionSubtitle} />
<div class="space-y-16"> { defaultProject?.projects.map((project, index) => ( <div class="card rounded-xl bg-secondary px-8 py-12 text-center shadow-lg md:grid md:grid-cols-2 md:place-items-center md:gap-4 md:rounded-tr-xl md:text-left lg:gap-8"> <div class={`py-12 ${index % 2 === 1 ? 'lg:order-2' : 'lg:order-1'}`} > <h4 class="relative mb-4 text-400 font-700 capitalize after:absolute after:left-[50%] after:top-[-.3rem] after:h-[6px] after:w-[55px] after:translate-x-[-50%] after:rounded-full after:bg-primary/80 md:after:left-6"> {project.name} </h4>
<p class="mb-8">{project.description}</p>
<div class="mb-8 flex flex-wrap items-center justify-center gap-4 md:justify-start"> {project.technologies?.map((tech) => ( <div class="flex items-center gap-2"> <img class="size-[30px]" src={tech.icon.asset.url} alt={tech.name} /> <li class="font-400 uppercase">{tech.name}</li> </div> ))} </div>
<div class="flex items-center justify-center gap-4 md:justify-start"> {project.button.map((item) => ( <Button asChild> <a href={item.url} target="_blank" rel="noopener noreferrer" class={`flex items-center gap-2 rounded-xl px-6 py-3 ${ item.icon === 'eye' ? 'bg-primary text-primary-foreground' : 'bg-card text-foreground' }`} > {item.icon === 'eye' ? ( <Eye size={20} /> ) : ( <Github size={20} /> )} <span class="font-500 capitalize">{item.title}</span> </a> </Button> ))} </div> </div>
<div class={`h-[320px] cursor-pointer overflow-hidden rounded-xl md:h-[352px] ${ index % 2 === 1 ? 'lg:order-1' : 'lg:order-2' }`} > <div class="card__img"> {project.imageType === 'upload' && checkImageExists(project.image?.asset.url) ? ( <Image class="h-full translate-y-0 rounded-xl object-cover duration-1000 ease-in-out" src={project.image?.asset.url as string} alt={project.image?.alt || 'project image'} background="blurhash" /> ) : project.imageType === 'url' && checkImageExists(project.url) ? ( <img class="h-full translate-y-0 rounded-xl object-cover duration-1000 ease-in-out" src={project.url} alt={project.image?.alt || 'project image'} /> ) : null} </div> </div> </div> )) } </div> </div></section>
<script> const cardImgs = document.querySelectorAll( '.card__img img' ) as NodeListOf<HTMLImageElement>;
cardImgs.forEach((img: HTMLImageElement) => { const card = img.closest('.card') as HTMLElement | null;
if (!card) { console.warn('Card element not found'); return; }
// Set initial transition properties img.style.transition = 'transform 2s cubic-bezier(0.4, 0, 0.2, 1)';
// Get initial dimensions const updateHoverEffect = () => { const imgHeight = img.offsetHeight; const cardHeight = card.offsetHeight;
// Mouse enter event img.addEventListener('mouseenter', () => { const percentageDifference = Math.abs( ((imgHeight - cardHeight) / imgHeight) * 105 ); img.style.transform = `translateY(-${percentageDifference}%)`; });
// Mouse leave event img.addEventListener('mouseleave', () => { img.style.transform = 'translateY(0)'; }); };
updateHoverEffect();
window.addEventListener('DOMContentLoaded', updateHoverEffect); });</script>
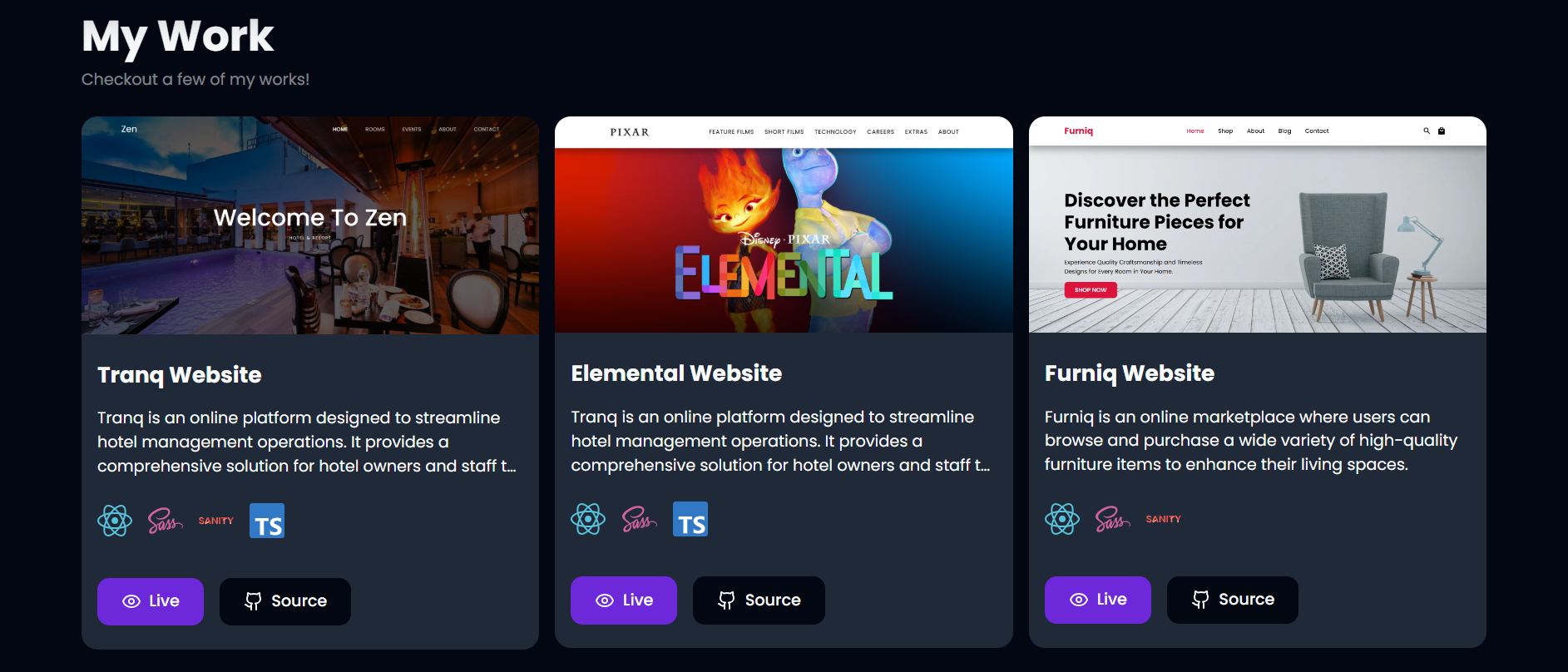
---import { getProjectContent } from '@/lib/queries';import { Button } from '@/components/ui/button';import { Eye, Github } from 'lucide-react';import { checkImageExists, getWorkByVariant } from '@/lib/helper';import Title from '@/components/Title';import { Image } from '@unpic/astro';
const projectsSection = await getProjectContent();
// Get specific hero variantconst defaultProject = getWorkByVariant(projectsSection, 'default');
if (!defaultProject) { throw new Error('Default hero variant not found in Sanity content');}---
<section id={defaultProject.id} class="pt-[10rem] md:pt-[7.5rem]"> <div class="container md:px-0"> <Title title={defaultProject.sectionTitle} subtitle={defaultProject.sectionSubtitle} alignment={defaultProject.titleAlignment} client:load />
<div class="space-y-10 md:grid md:grid-cols-2 md:gap-4 md:space-y-0 lg:grid-cols-3" > { defaultProject.projects.map((project) => ( <div> {project.imageType === 'upload' && checkImageExists(project.image?.asset.url) ? ( <Image class="h-[200px] w-full rounded-tl-2xl rounded-tr-2xl object-cover duration-1000 ease-in-out" src={project.image?.asset.url as string} alt={project.image?.alt} background="blurhash" /> ) : project.imageType === 'url' && checkImageExists(project.url) ? ( <img class="rounded-tl-2xl rounded-tr-2xl object-cover duration-1000 ease-in-out" src={project.url} alt={project.image?.alt} /> ) : null}
<div class="rounded-bl-2xl rounded-br-2xl bg-secondary px-4 py-6"> <h4 class="relative mb-4 text-300 font-700 capitalize"> {project.name} </h4> <p class="mb-6 line-clamp-3">{project.description}</p>
<div class="mb-2 flex flex-wrap gap-2"> <div class="mb-8 flex flex-wrap items-center justify-center gap-4 md:justify-start"> {project.technologies?.map((tech) => ( <div class="flex items-center gap-2"> <img class="size-[35px]" src={tech.icon.asset.url} alt={tech.name} /> </div> ))} </div> </div>
<div class="flex items-center justify-start gap-4"> {project.button.map((item) => ( <Button asChild> <a href={item.url} target="_blank" rel="noopener noreferrer" class={`flex items-center gap-2 rounded-xl px-6 py-3 ${ item.icon === 'eye' ? 'bg-primary' : 'bg-card' }`} > {item.icon === 'eye' ? ( <Eye size={20} /> ) : ( <Github size={20} /> )} <span class="font-500 capitalize">{item.title}</span> </a> </Button> ))} </div> </div> </div> )) } </div> </div></section>
How to Use
To implement these hero components:
- Copy the code from your desired hero variation
- Paste the code inside
Project.astro
file inside thesrc/sections
folder - Customize the content as needed through your Sanity Studio
The layout variants are designed to be flexible and work across different types of content while maintaining consistency in your design system.